Artificial Neural network (ANN) is an efficient computing system whose central theme is borrowed from the analogy of biological neural networks. ANNs are also named as Artificial Neural Systems, Parallel Distributed Processing Systems, and Connectionist Systems. ANN acquires large collection of units that are interconnected in some pattern to allow communications between them. These units, also referred to as nodes or neurons, are simple processors which operate in parallel.
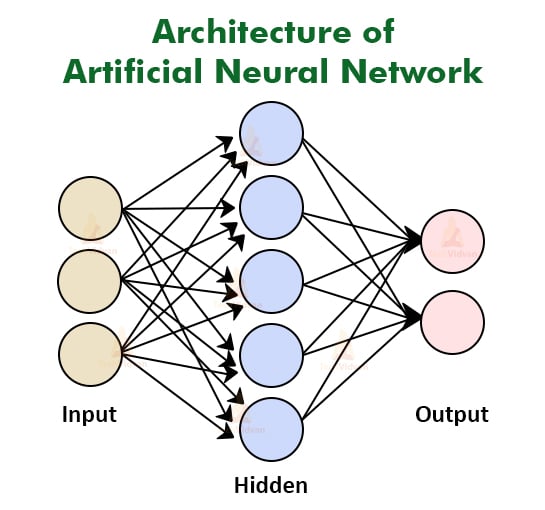
Every neuron is connected with other neuron through a connection link. Each connection link is associated with a weight having the information about the input signal. This is the most useful information for neurons to solve a particular problem because the weight usually excites or inhibits the signal that is being communicated. Each neuron is having its internal state which is called activation signal. Output signals, which are produced after combining input signals and activation rule, may be sent to other units.
For creating neural networks in Python, we can use a powerful package for neural networks called NeuroLab. It is a library of basic neural networks algorithms with flexible network configurations and learning algorithms for Python. You can install this package with the help of the following command on command prompt:
pip install NeuroLab
If you are using the Anaconda environment, then use the following command to install NeuroLab:
conda install -c labfabulous neurolab
Now let us build some neural networks in Python by using the NeuroLab package. Perceptrons are the building blocks of ANN. Following is a stepwise execution of the Python code for building a simple neural network perceptron based classifier:
Import the necessary packages as shown:
import matplotlib.pyplot as plt
import neurolab as nl
import neurolab as nl
Enter the input values. Note that it is an example of supervised learning, hence you will have to provide target values too.
input = [[0, 0], [0, 1], [1, 0], [1, 1]]
target = [[0], [0], [0], [1]]
target = [[0], [0], [0], [1]]
Create the network with 2 inputs and 1 neuron:
net = nl.net.newp([[0, 1],[0, 1]], 1)
Now, train the network. Here, we are using Delta rule for training.
error_progress = net.train(input, target, epochs=100, show=10, lr=0.1)
Now, visualize the output and plot the graph:
plt.figure()
plt.plot(error_progress)
plt.xlabel('Number of epochs')
plt.ylabel('Training error')
plt.grid()
plt.show()
plt.plot(error_progress)
plt.xlabel('Number of epochs')
plt.ylabel('Training error')
plt.grid()
plt.show()
You can see the following graph showing the training progress using the error metric:
In the next post we'll create a single layer neural network.
0 comments:
Post a Comment