Tic-Tac-Toe is very familiar and one of the most popular games. Let us create this game by using the easyAI library in Python.
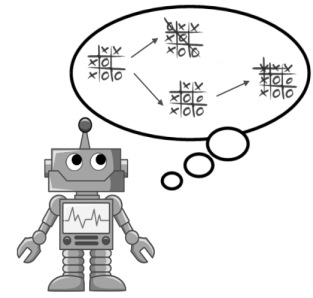
The following code is the Python code of this game:
from easyAI import TwoPlayersGame, AI_Player, Negamax
from easyAI.Player import Human_PlayerInherit the class from the TwoPlayerGame class to handle all operations of the game:
class TicTacToe_game(TwoPlayersGame):
def __init__(self, players):Now, define the players and the player who is going to start the game:
self.players = players
self.nplayer = 1Define the type of board:
self.board = [0] * 9
Now there are some certain things to define as follows:
Define possible moves:
def possible_moves(self):
return [x + 1 for x, y in enumerate(self.board) if y == 0]Define the move of a player:
def make_move(self, move):
self.board[int(move) - 1] = self.nplayerTo boost AI, define when a player makes a move:
def umake_move(self, move):
self.board[int(move) - 1] = 0Define the lose condition that an opponent have three in a line
def condition_for_lose(self):
possible_combinations = [[1,2,3], [4,5,6], [7,8,9],[1,4,7], [2,5,8], [3,6,9], [1,5,9], [3,5,7]]
return any([all([(self.board[z-1] == self.nopponent)
for z in combination]) for combination in possible_combinations])
for z in combination]) for combination in possible_combinations])
Define a check for the finish of game
def is_over(self):
return (self.possible_moves() == []) or self.condition_for_lose()
return (self.possible_moves() == []) or self.condition_for_lose()
Show the current position of the players in the game
def show(self):
print('\n'+'\n'.join([' '.join([['.', 'O', 'X'][self.board[3*j + i]]
for i in range(3)]) for j in range(3)]))
print('\n'+'\n'.join([' '.join([['.', 'O', 'X'][self.board[3*j + i]]
for i in range(3)]) for j in range(3)]))
Compute the scores.
def scoring(self):
return -100 if self.condition_for_lose() else 0
return -100 if self.condition_for_lose() else 0
Define the main method to define the algorithm and start the game:
if __name__ == "__main__":
algo = Negamax(7)
TicTacToe_game([Human_Player(), AI_Player(algo)]).play()
algo = Negamax(7)
TicTacToe_game([Human_Player(), AI_Player(algo)]).play()
You can see the following output and a simple play of this game:
. . .
. . .
. . .
Player 1 what do you play ? 1
Move #1: player 1 plays 1 :
O . .
. . .
. . .
Move #2: player 2 plays 5 :
O . .
. X .
. . .
. . .
Player 1 what do you play ? 1
Move #1: player 1 plays 1 :
O . .
. . .
. . .
Move #2: player 2 plays 5 :
O . .
. X .
. . .
Player 1 what do you play ? 3
Move #3: player 1 plays 3 :
O . O
. X .
. . .
Move #4: player 2 plays 2 :
O X O
. X .
. . .
Player 1 what do you play ? 4
Move #5: player 1 plays 4 :
O X O
O X .
. . .
Move #6: player 2 plays 8 :
O X O
O X .
. X .
Player 1 what do you play ? 3
Move #3: player 1 plays 3 :
O . O
. X .
. . .
Move #4: player 2 plays 2 :
O X O
. X .
. . .
Player 1 what do you play ? 4
Move #5: player 1 plays 4 :
O X O
O X .
. . .
Move #6: player 2 plays 8 :
O X O
O X .
. X .
0 comments:
Post a Comment